Using Sitecore Search in NextJS site
Expose data for indexing
The simplest way to use Sitecore Search is by crawling the website, in the Sitecore Search Introduction the various methods are presented.
You need to have the necessary information exposed on the site. NextJs have a neat <Head>
component that allows you to add meta tags to the head html tag, even from a component inserted on the page.
Example product.tsx
|
|
Configure indexer
You need configure the indexer in Sitecore Search to include the data in the desired attribute in the Document Extractors part.
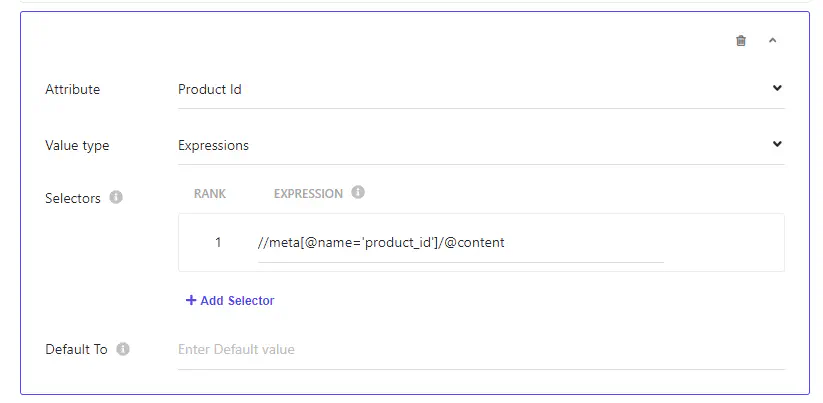
Components on pages
In Sitecore Search: React UI you can see how to use the SDK to generate client side components for search.
Search also provides a nice REST based api. With that you can make SSR and SSG pages based on search content.